On a project I’m currently working on, we use ActiveRecord callbacks heavily. From simplying creating other associated objects, to recording the historic activity about the object in question, and more.
The drawback I find from the scope of AR callbacks though is that you only have direct access to data from the database. And since you can’t pass arguments to a AR callback, you can’t easily get the request params for example or know which controller action fired off the the AR chain that triggered the callback you’re in, or know who the current user logged in is.
But it turns out that there is a way, however it is neither straight forward or obvious. Say for example you have the following model and controller:
1 16 end
1 12 end
What we want to accomplish here is that all admins of a group get a notification when another admin user edits a post. The post in question here has no context in it’s callback of which admin was logged in at the time the update happened. So what we’re gonna do is put the admin user’s id onto the current thread of the request in a before filter. Then, we’ll pull that id off in the callback to look up the admin and create the notifications.
So we’ll add a simple before filter:
1 7 end
And create a couple class methods on User to store the currently logged in admin for the duration of the request:
1 10 end
Now we can alter our note_activity method in Post to get the admin who did the edit:
1 2 group.admins.each do |admin| 3 admin.notifications.create(:user => User.current, 4 :action => 'did something') 5 end 6 end
This works well in my project. I’d like to know if there is a less “hacky” way of doing this but I’m not currently aware of one.
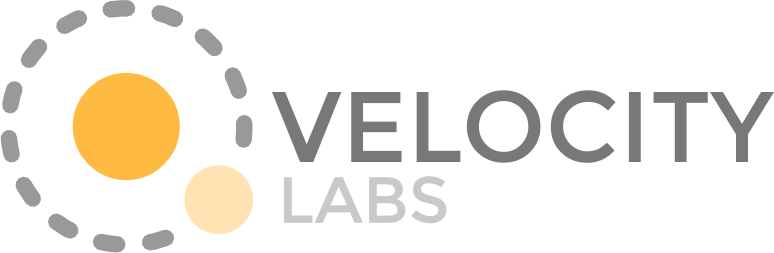
Need web application development, maintenance for your existing app, or a third party code review?
Velocity Labs can help.
{ 2 comments }